Algorithmic Trading Course: Step-by-Step Guide to Moving Average Crossover Strategy
- Bryan Downing
- 11 minutes ago
- 14 min read
Unlock Python for financial analysis with a downloadable, robust, and clearly explained trading strategy script.
Introduction: Python, Pandas, and the Algorithmic Trading CourseJourney
Python, powered by libraries like Pandas, has democratized algorithmic trading. Pandas DataFrames are invaluable for manipulating time-series data, essential for backtesting trading strategies. However, its intricacies, particularly around data modification, can lead to common pitfalls like the SettingWithCopyWarning, potentially derailing strategy development.
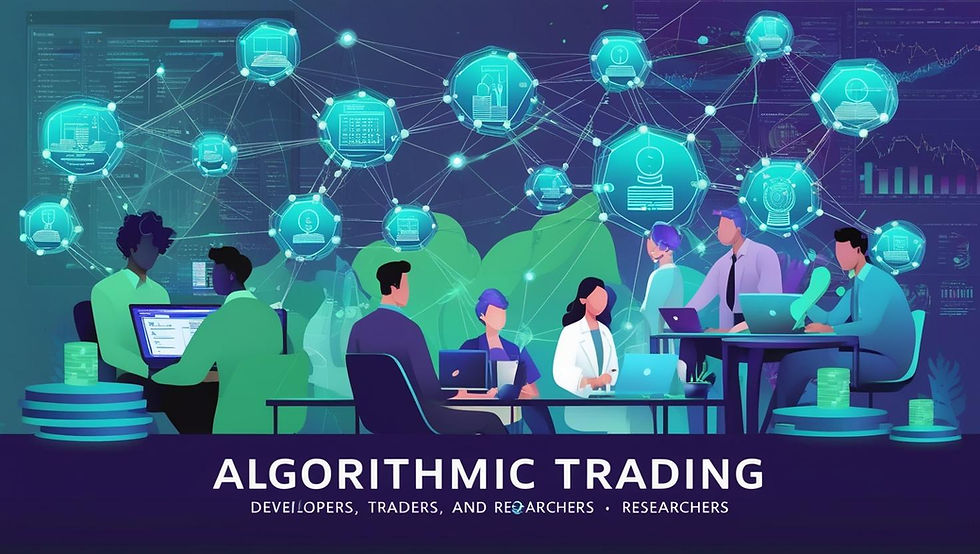
This article serves as a comprehensive guide, transforming a debugging process into a learning resource. We'll dissect common Pandas errors, explore best practices for indexing and assignment, and build a complete Moving Average (MA) Crossover trading strategy from the ground up. The focus is not just on providing code, but on explaining the why behind each step, ensuring you understand how to avoid common issues and write reliable financial analysis scripts.
You will gain:
A downloadable Python script for an MA Crossover strategy.
In-depth understanding of MA crossover logic, position management, and returns calculation.
Mastery of Pandas best practices, especially the use of df.loc for safe assignments.
Techniques for effective strategy visualization and dummy data generation for testing.
This guide is designed for anyone looking to solidify their Pandas skills for financial analysis, from novices to experienced developers seeking clarity on specific Pandas behaviors.
Get this Python script here
Chapter 1: Essential Pandas Concepts for Robust Strategies
Before building our strategy, let's cover crucial Pandas concepts that prevent common errors and ensure code reliability.
1.1. Required Libraries
Our script uses:
Pandas: For data manipulation (pip install pandas).
NumPy: For numerical operations (pip install numpy).
Matplotlib: For plotting (pip install matplotlib).
1.2. The SettingWithCopyWarning and the Power of .loc
The SettingWithCopyWarning is a frequent hurdle. It appears when Pandas is unsure if you're modifying the original DataFrame or a temporary copy, often due to chained indexing (e.g., df['column'][row] = value). If a copy is modified, the original DataFrame remains unchanged, leading to silent bugs.
The Solution: Single-Step Assignment with .locPandas recommends using .loc (for label-based access) or .iloc (for position-based access) for all assignments that modify a DataFrame. This provides an unambiguous instruction to Pandas:
df.loc[row_indexer, column_indexer] = value
This approach is central to our script, ensuring modifications are intentional and correct, thereby avoiding the SettingWithCopyWarning.
1.3. Views vs. CopiesPandas operations can return either a view (a direct reference to original data) or a copy (an independent duplicate). Modifying a view changes the original; modifying a copy does not. When unsure, or if you need an independent slice, use .copy(): new_df = df[condition].copy(). In our strategy, we'll start by copying the input data (df = data_input.copy()) to protect the original.
1.4. Class Structure for OrganizationWe'll use a Python class, MASlopeStrategy, to encapsulate our strategy's data (like MA periods) and functions (like calculating signals and plotting). This promotes organization, reusability, and maintainability.
Chapter 2: Anatomy of the MASlopeStrategy Script
Our MASlopeStrategy class will be structured as follows:
init(self, fast_ma_period=10, slow_ma_period=30): Initializes strategy parameters (MA periods) and self.signals (an empty DataFrame to hold all calculations).
calculatesignals_and_positions(self, data_input: pd.DataFrame): The core private method performing all calculations: MAs, signals, positions, and returns. This is detailed in Chapter 3.
visualize_strategy(self, symbol=""): Uses Matplotlib to plot prices, indicators, signals, positions, and cumulative returns.
run_strategy(self, data_input: pd.DataFrame, symbol="STOCK"): Orchestrates the process: calls calculations, then visualization.
if name == '__main__': block: Allows the script to be run directly, including dummy data generation for testing.
This structure provides a clear and modular framework.
Chapter 3: The Strategy's Core: calculatesignals_and_positions Explained
This method is the heart of our MA Crossover strategy, implementing the logic for signals, positions, and returns with Pandas best practices.
Python
# (Inside MASlopeStrategy class)
def calculatesignals_and_positions(self, data_input: pd.DataFrame) -> pd.DataFrame:
# 3.1 Input Validation and Data Copying
if not isinstance(data_input, pd.DataFrame) or data_input.empty or 'close' not in data_input.columns:
print("Warning: Input data is invalid, empty, or missing 'close' column.")
return pd.DataFrame()
df = data_input.copy()
# 3.2 MA Calculation
df['SMA_fast'] = df['close'].rolling(window=self.fast_ma_period, min_periods=self.fast_ma_period).mean()
df['SMA_slow'] = df['close'].rolling(window=self.slow_ma_period, min_periods=self.slow_ma_period).mean()
# 3.3 Signal Generation (Pinpointing Crossovers)
df['signal'] = 0.0 # Neutral signal
buy_condition = (df['SMA_fast'] > df['SMA_slow']) & (df['SMA_fast'].shift(1) <= df['SMA_slow'].shift(1))
df.loc[buy_condition, 'signal'] = 1.0 # Buy signal
sell_condition = (df['SMA_fast'] < df['SMA_slow']) & (df['SMA_fast'].shift(1) >= df['SMA_slow'].shift(1))
df.loc[sell_condition, 'signal'] = -1.0 # Sell signal
# 3.4 Iterative Position Logic (State Management)
df['position'] = 0.0 # Flat position
previous_position = 0.0
for i in range(len(df)):
current_index = df.index[i]
current_signal = df.loc[current_index, 'signal']
if pd.isna(df.loc[current_index, 'SMA_fast']): # Handle initial NaN period for MAs
df.loc[current_index, 'position'] = previous_position
continue
if current_signal == 1.0: # Enter long
df.loc[current_index, 'position'] = 1.0
elif current_signal == -1.0: # Enter short
df.loc[current_index, 'position'] = -1.0
else: # No new signal, maintain previous position
df.loc[current_index, 'position'] = previous_position
previous_position = df.loc[current_index, 'position']
df['position'] = df['position'].fillna(0.0)
# 3.5 Strategy Returns (Using Shifted Positions)
df['strategy_returns'] = df['close'].pct_change() * df['position'].shift(1)
if not df.empty: df.loc[df.index[0], 'strategy_returns'] = 0.0
df['strategy_returns'] = df['strategy_returns'].fillna(0.0)
# 3.6 Cumulative Strategy Returns
df['strategy_cumulative_returns'] = (1 + df['strategy_returns']).cumprod() - 1
if not df.empty: df.loc[df.index[0], 'strategy_cumulative_returns'] = 0.0
df['strategy_cumulative_returns'] = df['strategy_cumulative_returns'].fillna(0.0)
# 3.7 (Optional) Buy & Hold Benchmark
df['buy_hold_returns'] = df['close'].pct_change()
if not df.empty: df.loc[df.index[0], 'buy_hold_returns'] = 0.0
df['buy_hold_returns'] = df['buy_hold_returns'].fillna(0.0)
df['buy_hold_cumulative_returns'] = (1 + df['buy_hold_returns']).cumprod() - 1
if not df.empty: df.loc[df.index[0], 'buy_hold_cumulative_returns'] = 0.0
df['buy_hold_cumulative_returns'] = df['buy_hold_cumulative_returns'].fillna(0.0)
return df
Detailed Breakdown:
3.1 Input Validation and Data Copying: Ensures valid input and works on a df.copy() to prevent modifying the original data.
3.2 MA Calculation: Simple Moving Averages (SMA_fast, SMA_slow) are calculated using rolling().mean(). min_periods (equal to the window size) ensures SMAs are computed only with a full window of data, introducing initial NaNs which are handled.
3.3 Signal Generation:
'signal' column initialized to 0.0 (neutral).
Buy (1.0) and sell (-1.0) signals are generated when the fast SMA crosses the slow SMA. The conditions (df['SMA_fast'] > df['SMA_slow']) & (df['SMA_fast'].shift(1) <= df['SMA_slow'].shift(1)) (and its inverse for sells) precisely identify the crossover bar by comparing current SMA relationship with the previous bar's.
Assignments use df.loc[condition, 'signal'] = value.
3.4 Iterative Position Logic: This is crucial for correct state management.
'position' column initialized to 0.0 (flat). previous_position tracks the prior period's position.
A for loop iterates through the DataFrame.
If MAs are NaN (initial period), the previous_position (initially 0) is maintained.
If signal is 1.0 (buy), position becomes 1.0.
If signal is -1.0 (sell), position becomes -1.0.
If signal is 0.0 (no new crossover), the previous_position is maintained, correctly modeling holding a trade.
All position assignments use df.loc[current_index, 'position'] = ....
previous_position is updated for the next iteration.
3.5 Strategy Returns (strategy_returns):
Calculated as df['close'].pct_change() * df['position'].shift(1). This correctly uses the asset's price change and the position held before that change (from the previous day, via .shift(1)).
The first return and any other NaNs are filled with 0.0.
3.6 Cumulative Strategy Returns (strategy_cumulative_returns):
Calculated using (1 + df['strategy_returns']).cumprod() - 1, which compounds the periodic returns.
The first cumulative return is set to 0.0.
3.7 Buy & Hold Benchmark: Standard calculation for comparison.
This refined method ensures accurate calculation of all strategy components using clear logic and Pandas best practices.
Chapter 4: Visualization and Execution - Bringing the Strategy to Life
Effective visualization is key to understanding a strategy's behavior. The visualize_strategy method provides this, and the run_strategy method orchestrates the overall execution.
4.1. visualize_strategy Method Enhancements
This method uses matplotlib.pyplot for a multi-panel chart:
python
# (Inside MASlopeStrategy class)
def visualize_strategy(self, symbol: str = ""):
if self.signals.empty: # Guard clause
print(f"No signals data to visualize for {symbol}.")
return
fig, axes = plt.subplots(3, 1, figsize=(16, 12), sharex=True,
gridspec_kw={'height_ratios': [3, 1, 2]})
ax1, ax2, ax3 = axes[0], axes[1], axes[2]
fig.suptitle(f'MA Crossover Strategy Analysis: {symbol}', fontsize=16)
# Panel 1: Price, MAs, and Buy/Sell Signal Markers
ax1.plot(self.signals.index, self.signals['close'], label=f'{symbol} Close Price', ...)
# ... (plot SMAs) ...
# Enhanced: Plot buy/sell signal markers on the price chart
if 'signal' in self.signals.columns:
buy_idx = self.signals[self.signals['signal'] == 1.0].index
sell_idx = self.signals[self.signals['signal'] == -1.0].index
# ... (plot markers using ax1.plot(buy_idx, relevant_price_for_marker, '^', ...)) ...
ax1.set_title('Price, Moving Averages, and Trading Signals') # ... (other ax1 settings) ...
# Panel 2: Trading Position
if 'position' in self.signals.columns:
ax2.plot(self.signals.index, self.signals['position'], drawstyle='steps-post', ...)
# Enhanced: Descriptive y-axis labels for position
ax2.set_yticks([-1, 0, 1]); ax2.set_yticklabels(['Short', 'Neutral', 'Long'])
ax2.set_title('Trading Position Over Time') # ... (other ax2 settings) ...
# Panel 3: Cumulative Returns
if 'strategy_cumulative_returns' in self.signals.columns:
ax3.plot(self.signals.index, self.signals['strategy_cumulative_returns'] * 100, ...)
# Enhanced: Plot Buy & Hold cumulative returns for comparison
if 'buy_hold_cumulative_returns' in self.signals.columns:
ax3.plot(self.signals.index, self.signals['buy_hold_cumulative_returns'] * 100, ...)
ax3.set_title('Cumulative Returns Comparison') # ... (other ax3 settings) ...
plt.xlabel('Date'); plt.tight_layout(rect=[0, 0, 1, 0.96]); plt.show()
Multi-Panel Plot: Three stacked subplots for price/signals, position, and returns.
Panel 1 (Price & Signals): Shows close price, SMAs. Enhancement: Buy (^) and sell (v) markers are plotted at signal generation points for clarity.
Panel 2 (Position): Displays the position (1.0, -1.0, 0.0) using drawstyle='steps-post'. Enhancement: Y-axis labels are more descriptive.
Panel 3 (Cumulative Returns): Shows strategy cumulative returns. Enhancement: Includes Buy & Hold returns for benchmarking.
4.2. run_strategy Method
This orchestrates the workflow:
python
# (Inside MASlopeStrategy class)
def run_strategy(self, data_input: pd.DataFrame, symbol: str = "STOCK"):
print(f"\nRunning MA Crossover Strategy for {symbol}...")
self.signals = self._calculate_signals_and_positions(data_input)
if self.signals.empty or self.signals.isna().all().all(): # Check for valid output
print(f"Strategy calculation resulted in no valid signals for {symbol}. Skipping visualization.")
return
self.visualize_strategy(symbol=symbol)
print(f"Strategy run and visualization for {symbol} complete.")
4.3. if name == '__main__': Block (Dummy Data & Execution)This makes the script directly runnable for testing:
python
# (At the end of the script)
if name == '__main__':
# --- Dummy Data Generation ---
# Enhanced: More realistic dummy price data
num_days = 252 * 2; start_date = pd.to_datetime('2022-01-01')
dates = pd.date_range(start_date, periods=num_days, freq='B')
dummy_data_df = pd.DataFrame(index=dates)
daily_returns_sim = np.random.normal(loc=0.0005, scale=0.015, size=num_days)
dummy_data_df['close'] = 100 * (1 + daily_returns_sim).cumprod()
dummy_data_df['close'] = dummy_data_df['close'].round(2)
# --- Instantiate and Run ---
strategy = MASlopeStrategy(fast_ma_period=20, slow_ma_period=50)
strategy.run_strategy(data_input=dummy_data_df, symbol="DUMMY_STOCK")
# ... (Optional: print some summary metrics) ...
Dummy Data: Generates a more stock-like price series using np.random.normal for daily returns, allowing for better testing.
The strategy is instantiated and run, producing plots and console output.
Chapter 5: The Complete Python Script for Download
Below is the complete, commented Python script. Save it as ma_crossover_strategy.py and run it.
python
# ma_crossover_strategy.py
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# Optional: Configure Pandas for Copy-on-Write (recommended for future compatibility)
# pd.options.mode.copy_on_write = True
class MASlopeStrategy:
"""
Implements a Moving Average (MA) Crossover trading strategy.
Generates buy signals when a fast MA crosses above a slow MA,
and sell signals when the fast MA crosses below the slow MA.
"""
def init(self, fast_ma_period: int = 10, slow_ma_period: int = 30):
if not (isinstance(fast_ma_period, int) and isinstance(slow_ma_period, int) and
fast_ma_period > 0 and slow_ma_period > 0):
raise ValueError("MA periods must be positive integers.")
if fast_ma_period >= slow_ma_period:
raise ValueError("Fast MA period must be less than Slow MA period.")
self.signals = pd.DataFrame()
self.fast_ma_period = fast_ma_period
self.slow_ma_period = slow_ma_period
def calculatesignals_and_positions(self, data_input: pd.DataFrame) -> pd.DataFrame:
if not isinstance(data_input, pd.DataFrame) or data_input.empty or 'close' not in data_input.columns:
print("Warning: Input data for MA Crossover Strategy is invalid or missing 'close' column.")
return pd.DataFrame()
df = data_input.copy()
df['SMA_fast'] = df['close'].rolling(window=self.fast_ma_period, min_periods=self.fast_ma_period).mean()
df['SMA_slow'] = df['close'].rolling(window=self.slow_ma_period, min_periods=self.slow_ma_period).mean()
df['signal'] = 0.0
buy_condition = (df['SMA_fast'] > df['SMA_slow']) & (df['SMA_fast'].shift(1) <= df['SMA_slow'].shift(1))
df.loc[buy_condition, 'signal'] = 1.0
sell_condition = (df['SMA_fast'] < df['SMA_slow']) & (df['SMA_fast'].shift(1) >= df['SMA_slow'].shift(1))
df.loc[sell_condition, 'signal'] = -1.0
df['position'] = 0.0
previous_position = 0.0
for i in range(len(df)):
current_index = df.index[i]
current_signal = df.loc[current_index, 'signal']
if pd.isna(df.loc[current_index, 'SMA_fast']):
df.loc[current_index, 'position'] = previous_position
continue
if current_signal == 1.0: df.loc[current_index, 'position'] = 1.0
elif current_signal == -1.0: df.loc[current_index, 'position'] = -1.0
else: df.loc[current_index, 'position'] = previous_position
previous_position = df.loc[current_index, 'position']
df['position'] = df['position'].fillna(0.0)
df['strategy_returns'] = df['close'].pct_change() * df['position'].shift(1)
if not df.empty: df.loc[df.index[0], 'strategy_returns'] = 0.0
df['strategy_returns'] = df['strategy_returns'].fillna(0.0)
df['strategy_cumulative_returns'] = (1 + df['strategy_returns']).cumprod() - 1
if not df.empty: df.loc[df.index[0], 'strategy_cumulative_returns'] = 0.0
df['strategy_cumulative_returns'] = df['strategy_cumulative_returns'].fillna(0.0)
df['buy_hold_returns'] = df['close'].pct_change()
if not df.empty: df.loc[df.index[0], 'buy_hold_returns'] = 0.0
df['buy_hold_returns'] = df['buy_hold_returns'].fillna(0.0)
df['buy_hold_cumulative_returns'] = (1 + df['buy_hold_returns']).cumprod() - 1
if not df.empty: df.loc[df.index[0], 'buy_hold_cumulative_returns'] = 0.0
df['buy_hold_cumulative_returns'] = df['buy_hold_cumulative_returns'].fillna(0.0)
return df
def visualize_strategy(self, symbol: str = ""):
if self.signals.empty:
print(f"No signals data to visualize for {symbol}.")
return
fig, axes = plt.subplots(3, 1, figsize=(16, 12), sharex=True, gridspec_kw={'height_ratios': [3, 1, 2]})
ax1, ax2, ax3 = axes[0], axes[1], axes[2]
fig.suptitle(f'MA Crossover Strategy Analysis: {symbol}', fontsize=16)
ax1.plot(self.signals.index, self.signals['close'], label=f'{symbol} Close', color='black', alpha=0.9, lw=1.5)
if 'SMA_fast' in self.signals.columns:
ax1.plot(self.signals.index, self.signals['SMA_fast'], label=f'SMA {self.fast_ma_period}', color='dodgerblue', alpha=0.7, ls='--')
if 'SMA_slow' in self.signals.columns:
ax1.plot(self.signals.index, self.signals['SMA_slow'], label=f'SMA {self.slow_ma_period}', color='orangered', alpha=0.7, ls='--')
if 'signal' in self.signals.columns:
buy_idx = self.signals[self.signals['signal'] == 1.0].index
sell_idx = self.signals[self.signals['signal'] == -1.0].index
ref_series_for_markers = self.signals['SMA_slow'] if 'SMA_slow' in self.signals.columns else self.signals['close']
if not buy_idx.empty: ax1.plot(buy_idx, ref_series_for_markers.loc[buy_idx] * 0.99, '^', ms=10, color='green', label='Buy', alpha=0.8, lw=0)
if not sell_idx.empty: ax1.plot(sell_idx, ref_series_for_markers.loc[sell_idx] * 1.01, 'v', ms=10, color='red', label='Sell', alpha=0.8, lw=0)
ax1.set_ylabel('Price'); ax1.legend(loc='upper left'); ax1.grid(True, ls=':', alpha=0.6); ax1.set_title('Price, MAs & Signals')
if 'position' in self.signals.columns:
ax2.plot(self.signals.index, self.signals['position'], label='Position', color='purple', drawstyle='steps-post')
ax2.set_yticks([-1, 0, 1]); ax2.set_yticklabels(['Short', 'Neutral', 'Long'])
ax2.set_ylabel('Position'); ax2.legend(loc='upper left'); ax2.grid(True, ls=':', alpha=0.6); ax2.set_title('Trading Position')
if 'strategy_cumulative_returns' in self.signals.columns:
ax3.plot(self.signals.index, self.signals['strategy_cumulative_returns']*100, label='Strategy Returns', color='blue', lw=1.5)
if 'buy_hold_cumulative_returns' in self.signals.columns:
ax3.plot(self.signals.index, self.signals['buy_hold_cumulative_returns']*100, label='Buy & Hold', color='dimgray', ls='--')
ax3.set_ylabel('Cumulative Returns (%)'); ax3.legend(loc='upper left'); ax3.grid(True, ls=':', alpha=0.6); ax3.set_title('Cumulative Returns')
plt.xlabel('Date'); plt.tight_layout(rect=[0,0,1,0.96]); plt.show()
def run_strategy(self, data_input: pd.DataFrame, symbol: str = "STOCK"):
print(f"\nRunning MA Crossover Strategy for {symbol}...")
self.signals = self._calculate_signals_and_positions(data_input)
if self.signals.empty or self.signals.isna().all().all():
print(f"No valid signals for {symbol}. Skipping visualization.")
return
self.visualize_strategy(symbol=symbol)
print(f"Strategy run for {symbol} complete.")
if name == '__main__':
print("MA Crossover Strategy Demonstration")
num_days = 252 * 2; start_date = pd.to_datetime('2022-01-01')
dates = pd.date_range(start_date, periods=num_days, freq='B')
dummy_data_df = pd.DataFrame(index=dates)
daily_returns_sim = np.random.normal(loc=0.0005, scale=0.015, size=num_days)
dummy_data_df['close'] = 100 * (1 + daily_returns_sim).cumprod()
dummy_data_df['close'] = dummy_data_df['close'].round(2)
print(f"\nGenerated dummy data for {num_days} days. Sample (last 5):")
print(dummy_data_df.tail())
strategy = MASlopeStrategy(fast_ma_period=20, slow_ma_period=50)
strategy.run_strategy(data_input=dummy_data_df, symbol="DUMMY_STOCK_SMA_20_50")
if not strategy.signals.empty:
print("\n--- Summary (from generated signals DataFrame) ---")
print(strategy.signals[['close', 'SMA_fast', 'SMA_slow', 'signal', 'position',
'strategy_returns', 'strategy_cumulative_returns']].tail())
final_strat_ret = strategy.signals['strategy_cumulative_returns'].iloc[-1] * 100
final_bh_ret = strategy.signals['buy_hold_cumulative_returns'].iloc[-1] * 100
print(f"\nFinal Strategy Cumulative Return: {final_strat_ret:.2f}%")
print(f"Final Buy & Hold Cumulative Return: {final_bh_ret:.2f}%")
trades = strategy.signals[strategy.signals['signal'] != 0]['signal'].count()
print(f"Approximate trade signals generated: {trades}")
Chapter 6: Advancing Your Strategy - Next Steps and Considerations
This script provides a solid, understandable foundation. To build upon it for more realistic or complex scenarios, consider these areas:
6.1. Realism and Costs:
Transaction Costs: Incorporate brokerage fees and taxes into your return calculations. These can significantly impact profitability.
Slippage: Model the difference between expected and actual trade execution prices, especially for liquid assets or larger order sizes.
6.2. Sophistication:
Advanced Indicators: Explore RSI, MACD, Bollinger Bands, or combine multiple indicators for more robust signals.
Volume & Patterns: Use trading volume for signal confirmation or detect price patterns.
Risk Management: Implement position sizing rules (e.g., fixed fractional), stop-loss orders, and take-profit targets. This requires more complex position management logic.
6.3. Robustness and Testing:
Parameter Optimization: Systematically test different MA periods (or other strategy parameters) to find optimal settings, being wary of overfitting.
Walk-Forward Analysis: A more robust backtesting method than a single historical run, involving optimizing on one period and testing on a subsequent, unseen period, then shifting the window.
Out-of-Sample Testing: Always reserve a portion of your data that the strategy has never "seen" during development or optimization for a final, unbiased test.
6.4. Performance:
Vectorization: For very large datasets, explore fully vectorizing the position logic if the iterative approach becomes a bottleneck. This often involves clever use of ffill(), where(), and boolean masks, but can reduce readability.
6.5. Pandas Copy-on-Write (CoW):
Consider enabling Pandas' Copy-on-Write mode (pd.options.mode.copy_on_write = True) for more predictable behavior with DataFrame modifications, aligning with future Pandas defaults.
Conclusion: Building a Solid Foundation in Algorithmic Trading
This article has guided you through creating a Moving Average Crossover trading strategy in Python, with a strong emphasis on using Pandas correctly and understanding the logic behind each calculation. By focusing on clear signal generation, meticulous iterative position management, accurate returns calculation using .loc for assignments, and informative visualizations, you've gained a practical toolkit and a deeper understanding of common challenges.
The provided script is more than just code; it's a launchpad for your explorations into quantitative finance. Experiment with it, extend its capabilities, and continue to learn. The principles of careful data handling, logical state management, and robust testing are universal in building successful algorithmic trading systems. With this foundation, you are better equipped to navigate the exciting and complex world of data-driven trading.
Comments