How to create free AI agents: Research Report in Python
- Bryan Downing
- Feb 3
- 4 min read
Artificial intelligence is rapidly evolving beyond simple chatbots and image generators. The next frontier is the development of AI agents – autonomous entities capable of planning, reasoning, and executing complex tasks. These agents, guided by structured workflows, represent a significant leap forward in AI capabilities, and many believe 2025 will be the year they truly take center stage. This is how to create Free AI Agents. This article provides a practical example, demonstrating how to build an AI agent in Python that can research and write a report on a given topic. We'll use the example of DeepSeek R1's announcement and its impact on Nvidia investors as a case study. This is one example on how to create free AI agents.
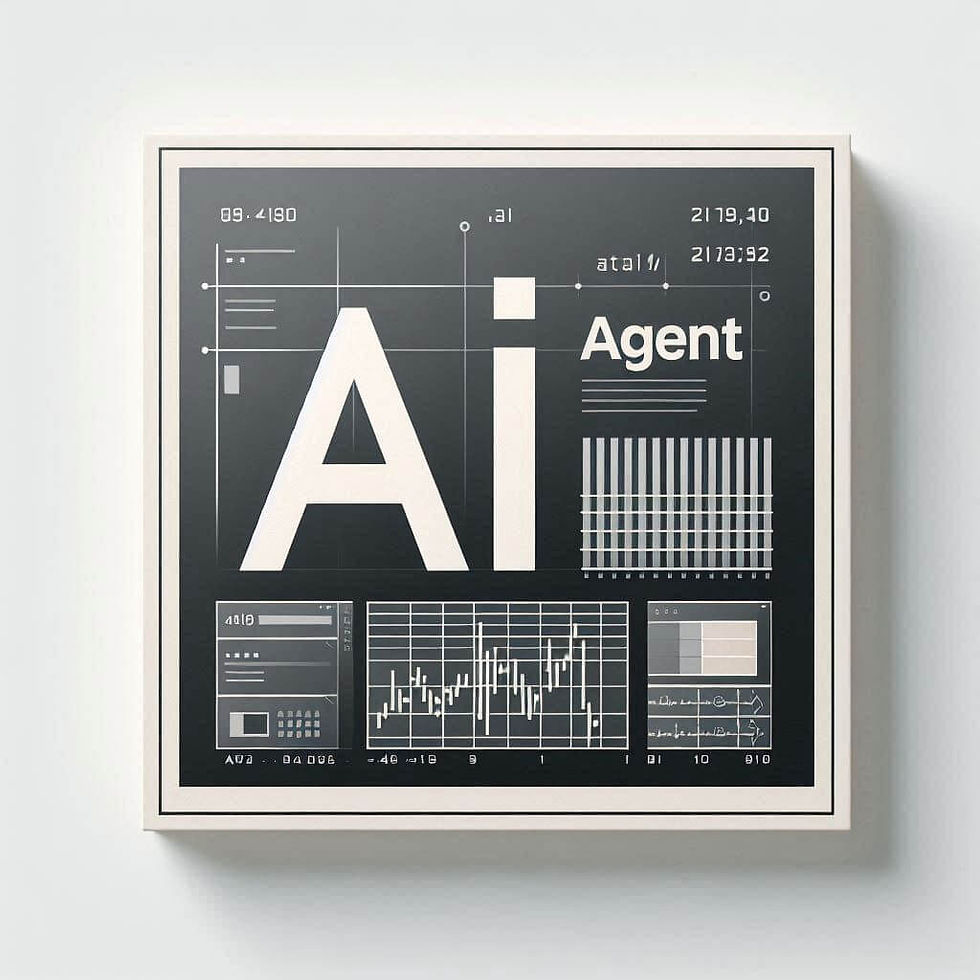
The core concept behind AI agents is the agentic workflow. This involves breaking down a complex goal into a series of smaller, manageable steps. Each step is executed by the AI, guided by carefully crafted prompts that provide context, instructions, and constraints. These prompts are the key to directing the AI's reasoning and ensuring it stays on track.
Our research report agent will follow a workflow like this:
Topic Definition: The agent receives the topic: "Why did the announcement of DeepSeek R1 rattle investors in Nvidia on January 27?"
Information Gathering: The agent uses search engines and other online resources to gather relevant information about DeepSeek R1, Nvidia's stock performance, and investor sentiment around January 27th. This might involve querying APIs, scraping websites, or using specialized research tools.
Analysis and Synthesis: The agent analyzes the gathered information, identifying key trends, relationships, and arguments. It synthesizes this information into a coherent narrative.
Report Generation: The agent generates a structured research report, including an introduction, background information, analysis of the impact of DeepSeek R1, discussion of investor sentiment, and a conclusion.
Let's look at a simplified Python implementation of this agent:
Python
import os
# You'll need to install these libraries:
# pip install google-search-results langchain transformers
from langchain.llms import OpenAI # Or another LLM
from langchain.agents import initialize_agent, Tool
from langchain.agents import AgentType
from langchain.tools import GoogleSearchAPIWrapper
# Set your OpenAI API key
os.environ["OPENAI_API_KEY"] = "YOUR_OPENAI_API_KEY"
# Set your SerpAPI key if using Google Search API
os.environ["SERPAPI_API_KEY"] = "YOUR_SERPAPI_API_KEY"
# Initialize LLM
llm = OpenAI(temperature=0) # Temperature controls creativity
# Initialize search tool
search = GoogleSearchAPIWrapper()
tools = [
Tool(
name = "Search",
func=search.run,
description="useful for when you need to answer questions about current events or the current state of the world. You should ask targeted questions"
)
]
# Initialize agent
agent = initialize_agent(tools, llm, agent=AgentType.ZERO_SHOT_REACT_DESCRIPTION, verbose=True)
# Define the topic
topic = "Why did the announcement of DeepSeek R1 rattle investors in Nvidia on January 27?"
# Run the agent
report = agent.run(f"Write a research report on: {topic}")
# Print the report
print(report)
Explanation:
Libraries: The code uses langchain, a powerful library for building LLM applications, along with tools for search. You'll need to install these libraries.
LLM Initialization: We initialize an OpenAI language model (you can replace this with other LLMs like those available through Hugging Face).
Search Tool: We use GoogleSearchAPIWrapper (or you can use other search tools) to enable the agent to access external information. You will need a SerpAPI key.
Agent Initialization: We initialize the agent with the LLM, the search tool, and the ZERO_SHOT_REACT_DESCRIPTION agent type, which allows the agent to decide which tool to use based on the description. The verbose=True setting provides detailed output of the agent's thought process.
Topic Definition: We define the research topic.
Agent Execution: We run the agent with the topic, prompting it to write a research report.
This is a simplified example. A more sophisticated agent might include:
Multiple Tools: The agent could have access to other tools, such as a news API, a financial data API, or even a code interpreter for analyzing data.
Memory: The agent could retain information from previous steps, allowing it to build upon its knowledge and avoid redundant searches.
Prompt Engineering: Carefully crafted prompts are crucial for guiding the agent's reasoning. Prompts can be designed to elicit specific types of information, constrain the agent's responses, and encourage critical thinking.
Output Formatting: The agent could be instructed to generate the report in a specific format, such as Markdown or HTML.
The example code provides a starting point for building your own AI agents. By expanding the agent's capabilities and refining its workflow, you can create powerful tools for research, analysis, and report generation.
The development of AI agents is still in its early stages, but the potential is enormous. As AI technology continues to advance, we can expect to see even more sophisticated agents capable of handling increasingly complex tasks. The year 2025 may indeed be the year of the agent, marking a turning point in the evolution of artificial intelligence. By understanding the principles behind agentic workflows and experimenting with tools like Langchain, you can be at the forefront of this exciting new era.
Opmerkingen